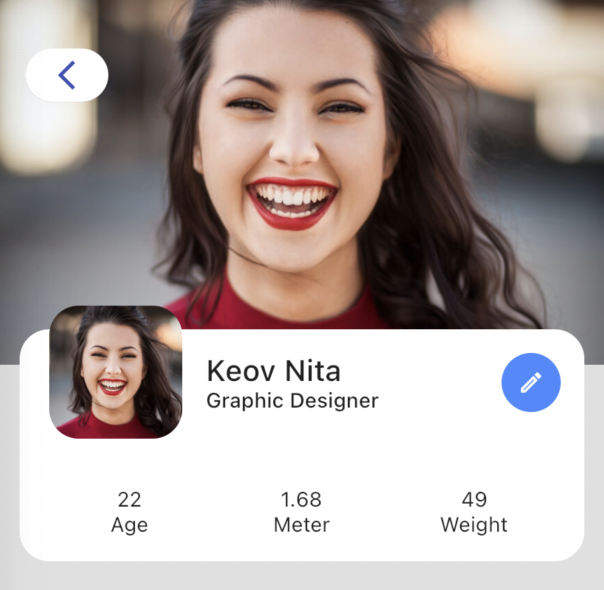
Flutter user profile page – Flutter modern profile ui with custom layout – free source code
- admin
- 0
- on Sep 11, 2022
We use flutter language to create this modern user profile page, in this flutter profile page you will learn many widget such as;
- Stack
- SingleChildScrollView
- CircleAvata
- Position
- Expanded
- …
User Profile Page Sample

Source Code
- ProfilePage9.dart
import 'package:flutter/material.dart';
class ProfilePage9 extends StatelessWidget {
const ProfilePage9({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.grey.shade300,
body: SingleChildScrollView(
child: Stack(
children: [
SizedBox(
height: 280,
width: double.infinity,
child: Image.network(
'https://romanticlovesms.com/wp-content/uploads/2022/03/Funny-Captions-for-Profile-Picture-1.jpg',
fit: BoxFit.cover,
),
),
Container(
margin: EdgeInsets.fromLTRB(16.0, 240.0, 16.0, 16.0),
child: Column(
children: [
Stack(
children: [
Container(
padding: EdgeInsets.all(16.0),
margin: EdgeInsets.only(top: 16.0),
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(20.0)),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Container(
margin: const EdgeInsets.only(left: 110.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Column(
crossAxisAlignment:
CrossAxisAlignment.start,
mainAxisAlignment:
MainAxisAlignment.start,
children: [
Text(
"Keov Nita",
style: Theme.of(context)
.textTheme
.headline6,
),
Text(
"Graphic Designer",
style: Theme.of(context)
.textTheme
.bodyText1,
),
SizedBox(
height: 40,
)
],
),
Spacer(),
CircleAvatar(
backgroundColor: Colors.blueAccent,
child: IconButton(
onPressed: () {},
icon: Icon(
Icons.edit_outlined,
color: Colors.white,
size: 18,
)),
)
],
)),
SizedBox(height: 10.0),
Row(
children: [
Expanded(
child: Column(
children: const [Text("22"), Text("Age")],
),
),
Expanded(
child: Column(
children: const [Text("1.68"), Text("Meter")],
),
),
Expanded(
child: Column(
children: const [Text("49"), Text("Weight")],
),
),
],
),
],
),
),
Container(
height: 90,
width: 90,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(20.0),
image: const DecorationImage(
image: NetworkImage(
'https://romanticlovesms.com/wp-content/uploads/2022/03/Funny-Captions-for-Profile-Picture-1.jpg'),
fit: BoxFit.cover)),
margin: EdgeInsets.only(left: 20.0),
),
],
),
const SizedBox(height: 20.0),
Container(
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(20.0),
),
child: Column(
children: const [
ListTile(
title: Text("Profile Information"),
),
Divider(),
ListTile(
title: Text("Date of Birth"),
subtitle: Text("12 September 2001"),
leading: Icon(Icons.calendar_view_day),
),
ListTile(
title: Text("Education"),
subtitle: Text("Bachelor Degree"),
leading: Icon(Icons.school_outlined),
),
ListTile(
title: Text("Email"),
subtitle: Text("nita.khgd@gmail.com"),
leading: Icon(Icons.mail_outline),
),
ListTile(
title: Text("Phone"),
subtitle: Text("+855 12 987 789"),
leading: Icon(Icons.phone),
),
ListTile(
title: Text("Address"),
subtitle: Text(
"Stung Meanchey District, Stung Meanchey Commune, Phnom Penh, Cambodia 1200"),
leading: Icon(Icons.map),
),
SizedBox(
height: 10,
),
],
),
)
],
),
),
Positioned(
top: 60,
left: 20,
child: MaterialButton(
minWidth: 0.2,
elevation: 0.2,
color: Colors.white,
child: const Icon(Icons.arrow_back_ios_outlined,
color: Colors.indigo),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(30.0),
),
onPressed: () {},
),
),
],
),
),
);
}
}