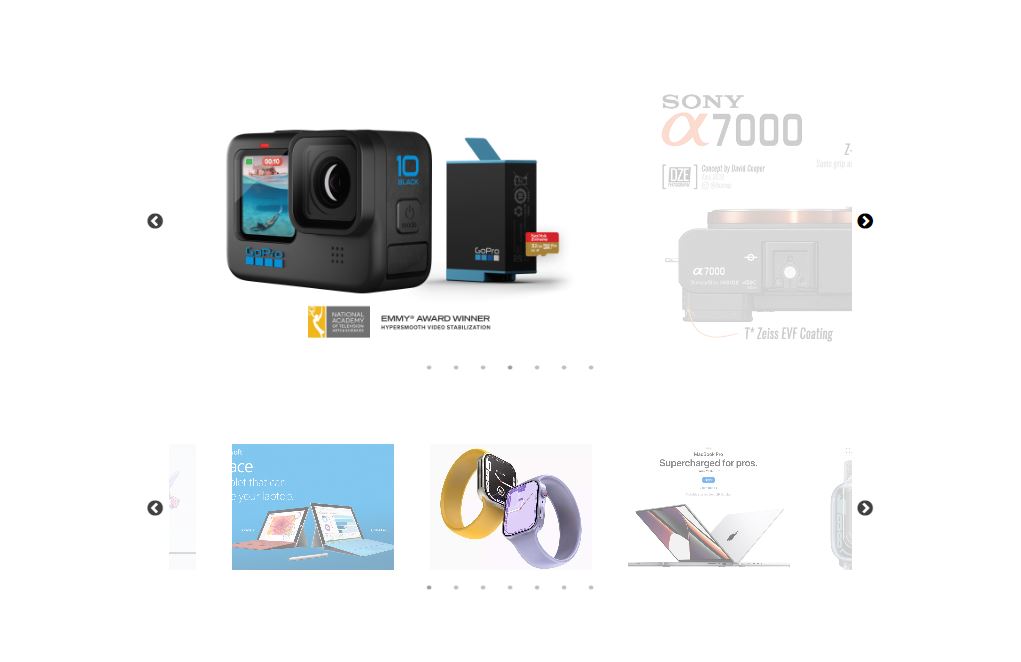
Image slider with Jquery Slick and PHP
- admin
- 0
- on Sep 22, 2022
In this article, we will create a dynamic image slider with Jquery Slick and PHP. To create a dynamic image slider we need to use PHP serverside script to query data from MySQL. The first file we need is a connection.php
- connection.php
<?php
$servername = "localhost";
$username = "root";
$password = "";
$db = "khodecam";
// Create connection
$conn = new mysqli($servername, $username, $password,$db);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
//echo "Connected successfully";
?>
The second file is index.php
2. index.php
<?php
include 'connection.php';
?>
<!DOCTYPE html>
<html>
<head>
<title>Khodecam Image Slider</title>
<meta charset="UTF-8">
<link rel="stylesheet" type="text/css" href="./slick/slick.css">
<link rel="stylesheet" type="text/css" href="./slick/slick-theme.css">
<style type="text/css">
html, body {
margin: 0;
padding: 0;
}
* {
box-sizing: border-box;
}
.slider {
width: 50%;
margin: 100px auto;
}
.slick-slide {
margin: 0px 20px;
}
.slick-slide img {
width: 100%;
}
.slick-prev:before,
.slick-next:before {
color: black;
}
.slick-slide {
transition: all ease-in-out .3s;
opacity: .2;
}
.slick-active {
opacity: .5;
}
.slick-current {
opacity: 1;
}
.web_banner{
object-fit: cover;
height:290px
}
.web_banner_small{
object-fit: cover;
height:140px
}
</style>
</head>
<body>
<section class="variable slider">
<?php
$sql = "SELECT * FROM tbl_product";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
?>
<div>
<img class="web_banner" src="<?php echo $row['proThumbnail'] ?>">
</div>
<?php
}
} else {
echo "0 results";
}
?>
</section>
<section class="center slider">
<?php
$sql = "SELECT * FROM tbl_product";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
?>
<div>
<img class="web_banner_small" src="<?php echo $row['proThumbnail'] ?>">
</div>
<?php
}
} else {
echo "0 results";
}
?>
</section>
<script src="https://code.jquery.com/jquery-2.2.0.min.js" type="text/javascript"></script>
<script src="./slick/slick.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript">
$(document).on('ready', function() {
$(".center").slick({
dots: true,
infinite: true,
centerMode: true,
slidesToShow: 3,
slidesToScroll: 3
});
$(".variable").slick({
dots: true,
infinite: true,
variableWidth: true
});
});
</script>
</body>
</html>
Third, we need an SQL script
3. khodecam.sql
CREATE DATABASE IF NOT EXISTS `khodecam`
USE `khodecam`;
CREATE TABLE IF NOT EXISTS `tbl_product` (
`proId` int(11) NOT NULL AUTO_INCREMENT,
`proName` char(150) DEFAULT NULL,
`proPrice` double DEFAULT NULL,
`proDetail` varchar(50) DEFAULT NULL,
`proStatus` int(11) DEFAULT 1,
`proThumbnail` varchar(250) DEFAULT NULL,
PRIMARY KEY (`proId`)
) ENGINE=InnoDB AUTO_INCREMENT=8 DEFAULT CHARSET=utf8mb4;
INSERT INTO `tbl_product` (`proId`, `proName`, `proPrice`, `proDetail`, `proStatus`, `proThumbnail`) VALUES
(1, 'iWatch8', 900, NULL, 1, 'https://economictimes.indiatimes.com/thumb/msid-92667788,width-1200,height-900,resizemode-4,imgsize-29322/apple-watch-series-8.jpg?from=mdr'),
(2, 'MacBook Pro 14', 2500, NULL, 1, 'https://i.gadgets360cdn.com/large/macbook_pro_2021_india_availability_image_apple_1635230071675.jpg'),
(3, 'iWatch7', 400, NULL, 1, 'https://static-01.daraz.lk/p/81871d1fd9865874c2f261b000da7b65.jpg'),
(4, 'GoPro10', 1800, NULL, 1, 'https://static.gopro.com/assets/blta2b8522e5372af40/blt6fbe24e08b6ebc0d/62d807e8d9851110d46cf775/pdp-h10-q2-july-25th-sa-gallery-1920-2x.png'),
(5, 'Sony Camera', 2000, NULL, 1, 'https://www.sonyalpharumors.com/wp-content/uploads/2018/04/A70001.jpg'),
(6, 'Ipad', 1800, NULL, 1, 'https://media.idownloadblog.com/wp-content/uploads/2020/04/iPad-Pro-float-ad-banner.jpg'),
(7, 'Surface', 3000, NULL, 1, 'https://www.edu365.ky/images/2018/11/29/surface-hero-banner.jpg');
Result
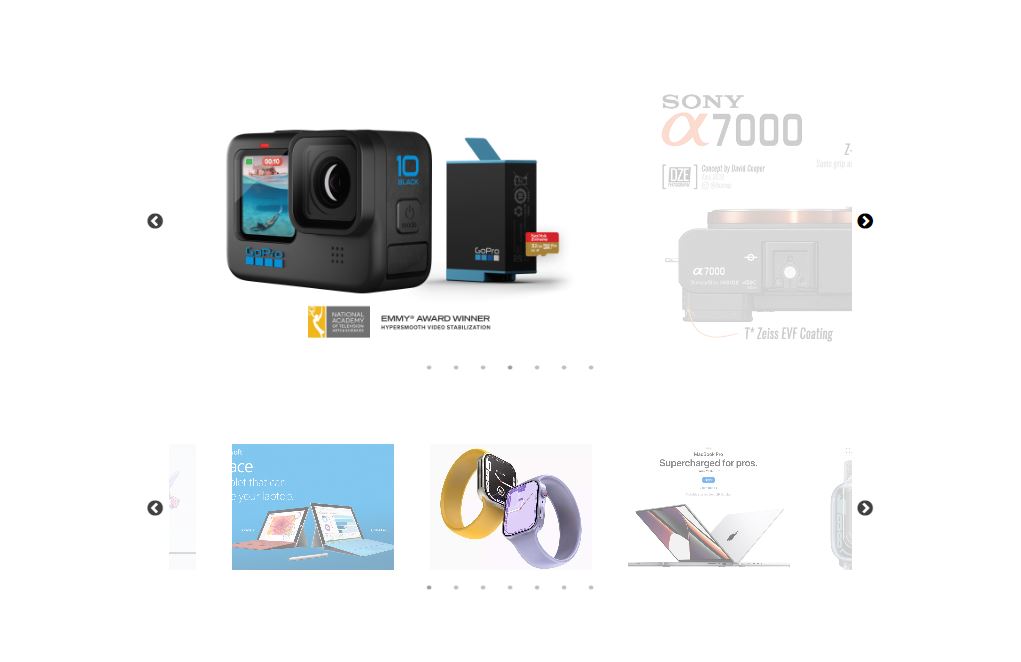
You can download the full source code here
Hi there, I enjoy reading all of your post. I like to write a little comment to support you.